Unit Tests are your Salvation
You are not smart enough to understand the totality of your change set
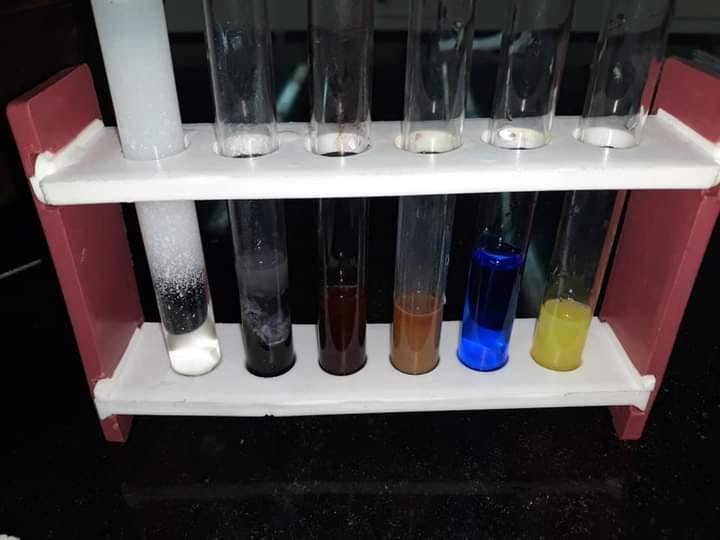
Picture a small CRUD application with some basic business logic in the backend that interacts with a database. You have a known bug in one of the methods and need to push a fix as soon as possible for your end-users. After a few hours of testing and debugging, the change ends up as only one line. This gives you the confidence to push the change after local interactive testing passes your checklist. The error was removed and now users have the original behavior in place.
Shortly after, your inbox floods with user complaints that they are unable to update existing data in the application. You are confident that your previous change had nothing to do with the new error, so you spend hours debugging again, trying to figure out what the issue is. After struggling, yelling, and swearing, you finally decide to go back and revert your changes and confirm that the issue is now gone. The single line of code was in an unrelated area of the application, how did it suddenly break part of the business functionality?
If this story sounds familiar, it is due to almost every developer having this scenario happen to them in some part of their career. We try to pretend we are smart enough to understand the totality of our code, the totality of the given inputs, and the totality of the given outputs, and combine all this knowledge to predict if the application is satisfying the business use cases. But this is a flawed approach, for as much as we can try to predict the future, second and third-order effects are often hard to grasp and understand easily. The average person can memorize a simple set of numbers up to a count of 7 without mistakes. Now apply that idea to thousands of lines of code. This effort becomes impossible once a codebase becomes sufficiently complex, which is an extremely low barrier to reach. The simple truth is we do not have enough brainpower to flawlessly understand the changes to a program. We need not fight this limitation and instead learn to work around it. This is where rigorous unit testing of an application can spot flaws in an implementation far sooner than a human can.